#4 Assignment - Inter-Process Communication
Question 1
Write a program in C programming language using IPC primitives (shared memory or pipe) to satisfy the described following problem:
- The parent process creates eight children processes which are waiting for a data structure to assign. These processes are called Processing Units (PU).
- The parent process should generate the first hundred prime numbers, and store them in a struct which has a value and a counter attribute (value equals to counter at the beginning), then assign these structures to children based on the modular division of the prime number by 8.
- These structs are assigned to the PUs one by one, in a concurrent way.
- The PUs add their corresponding numbers (0 to 7) to the value and decrement the counter, and then pass the struct to next PU. This loop continues until the counter is zero.
- When the counter is zero, the PU passes the struct to the parent and parent prints it out.
struct prime {
int value; // value of the prime number
int counter; // a counter to decrement (at the start counter = value)
}
An example scenario is illustrated in the following figure.
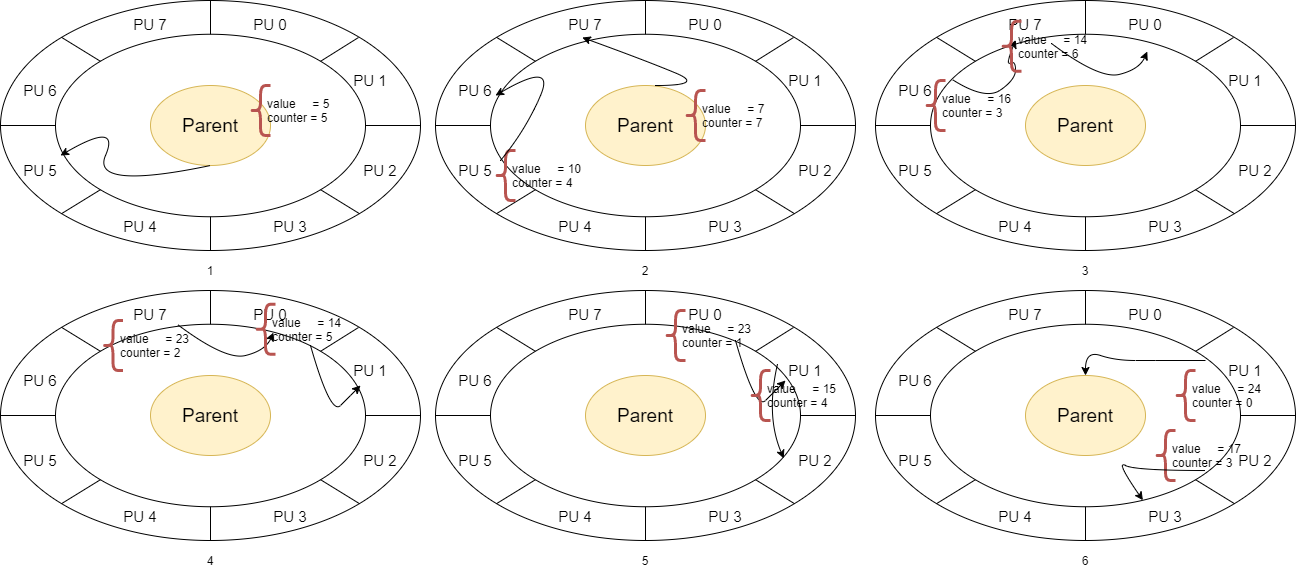
In your implementation, you are free to use either pipe or shared memory or semaphores or a combination of these. This brings an expectation about submitting different codes. :DD
Question 2
Write a report about the following subjects:
- The difference between Cross Memory Attach and other models of IPC methods introduced in the class.
- The safety of the pipe mechanism.
- Can
pthread_mutex_lock
be shared among processes (just like semaphores) instead of threads? Why or why not?
Question 3
Cross Memory Attach (CMA) is one of the models for Inter-Process Communication.
The system calls process_vm_read()
and
process_vm_write()
are based on this model.
Assume one of the two following scenarios based on your student number and implement
two C programs to satisfy the desired scenario.
The students with odd student number should consider the first scenario and the others consider second.
- A producer program should produce 3 Gigabytes data. A consumer should read that data and prints out.
- A producer produces 3 Gigabytes of data and then writes in the consumer's memory space.
Note that to synchronize the producer with the consumer you can use either
getchar()
or sleep()
.
Also, recall memset()
from the class session to generate data.
Some reading materials are:
https://man7.org/linux/man-pages/man2/process_vm_readv.2.html
https://lwn.net/Articles/405284/
https://stackoverflow.com/questions/17324564/cross-memory-attach-how-do-i-get-the-remote-address-from-a-child-process-to-a-p
Deadline
Your midterm is considered for determining the deadline.
- Tuesday 8th Dec. 23:00
Submission
Submit just a zip file, containing your codes and a report in PDF, in LMS. The file should be named as
[9752xxxx.zip
].
For example 97521234.zip
.